What Is Python?
Python is an interpreted programming language.It is object-oriented high-level programming language.Compared to other programming languages it is highly readable and has less syntax elements.
How Python applications are executed?
At runtime Python programs are executed by the interpreter.So you have to install the Python interpreter on your machine if you want to execute Python programs.You don’t need to compile your program ,it is directly executed by the interpreter.Internally it converts the source code to bytecode before it is converted to machine code.
Is Python Object Oriented?
Yes Python is Object Oriented.You can write programs in Python which follows the OOP concepts of Encapsulation,Abstraction,Polymorphism and Inheritance.
What is a List?
List in Python is a collection of values.You define list as:
my_list = [1, 2, 3]
you can even declare different types of data in a List
my_list = [1, 2, “three”]
What are the advantages of Python?
- Easy syntax to learn
- Dynamic
- Object-oriented
- Extensible
What are built in data types in Python?
- boolean
- int: Integers
- long: Long integers
- float: Floating Point numbers
- complex: Complex Numbers
- str: String
- bytes: integers in the range of 0-255
- byte array: byte array
- list
- tuple
Is python statically or dynamically typed?
Python is dynamically typed as you don’t need to assign data type to variables when you declare them
For example you can declare a variable as:
name=1 and then
reassign name with string value :
x=”Python”
What are the different loops in Python?
Two of the most common looping structures in Python are:
While loop
while (boolean expression): ... loop_body
For loop
for i in range (1,5): loop_body

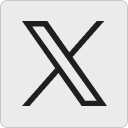
Leave a Reply