Web applications have a different execution model from the thick client or windows applications.Unlike windows applications which executes locally web applications executes on the remote server while user interaction with the application happens on the client’s browser.So the webpage needs to post data to the server to update the information on the server.But sometimes we need to store the data locally on the client’s machine.This has always been a big challenge for the web applications.
This is because HTTP is a stateless protocol which means that once the current request is completed all the state information is lost.
When the web page needs to access data ,it needs to fetch the data from the web server.This impacts the performance as there is a network latency in the case of web applications.Also this results in server load as the webserver needs to fetch and return the data.
To overcome these limitations client storage is used in the case of web applications.Web Storage is one such technology provided by HTML5 which supports client side storage
Some of the advantages of client side storage are:
1)Improved performance : as we don’t need to wait for the response from the web server.The data can be displayed as soon as the user clicks on a button.
2)Application can work Offline: Our application can work even when it is offline ,the data can be synced back with the web server once the network connection is available.
But one precaution which needs to be taken when storing the data locally on the client is since the data is more vulnerable as it is stored on the client instead of server so it needs to be ensured that it is not tampered with.
Cookies were used for storing data on the client but HTML5 webstorage provides the following adavantages
- Cookies are transmitted with every HTTP request,while webstorage doesn’t needs to be transmitted to the server for every request.
- Cookies have a size limit of 4KB,while webstorage provides 5MB size limit in the case of most browsers.
Webstorage depending upon the usage can be of two types.
- Local Storage: stores the data with no expiration and data is available even after the session expires.
- Session Storage: stores data for a specific session
There are two different objects localStorage and sessionStorage available in javascript for these two storage types
We can check if the browser supports web storage using the following code
if(typeof(Storage) !== "undefined") { // browser supports web storage } else { //browser does not support web storage }
We can also use Modernizr library to detect if our browser supports localstorage
if (Modernizr.localstorage) { //localStorage is supported } else { //localStorage is not supported }
Two important methods defined by the LocalStorage and SessionStorage objects are:
- setItem(“key”, “value”)
- getItem(“key”)
If our browser supports webstorage we can set the data in the localStorage using the following code
localStorage.setItem("key", "value"); or localStorage.key=value
for retrieving we can use the following
localStorage.getItem("key"); or localStorage.key
We can remove item which we have previously stored using the removeItem() method as:
localStorage.removeItem(“key”)
Similarly we can use the sessionStorage.To use sessionStorage we just need to replace localStorage with sessionStorage in the above code.The syntax for both LocalStorage and SessionStorage is same though each is used in a different scenario.
In chrome to view the LocalStorage and SessionStorage currently being used click on the developer tools.In the resources tab we can view the SessionStorage and LocalStorage being used.
Web Storage in HTML5 provides an efficient way of storing information on the client.

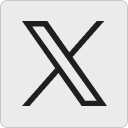
Leave a Reply