JSON is a data exchange format which is commonly used to exchange the data between the web server and the web browser.In Fetching json data using jquery ajax in MVC we looked at how to fetch data using JSON from the MVC controller.Now we will see how to pass data in JSON format from the web browser to the controller action method.
In the following example user will be entering data in a html table.The data will posted to the action method in the JSON format.The action method parameter will be populated with the posted JSON objects ,using the built in model binding.Once we have the collection of objects in the action method we can perform an operation on it such as saving in the database.
We will be sending the following object using json from the view to the action method.
public class Employee { public string Name { get; set; } public string Address { get; set; } public string PhoneNo { get; set; } public string Country { get; set; } }
In the view we are creating an html table having multiple rows.Each row has different cells corresponding to the Employee class properties such as Name,Address and Phone number.
<table id="tblEmployees"> <tr> <td >Name</td> <td ><input id="name" type="text" /></td> <td >Address</td> <td><input id="address" type="text" /></td> <td >Phone number</td> <td><input id="phone" type="text" /></td> <td >Country</td> <td><input id="country" type="text" /></td> </tr> <tr> <td>Name</td> <td><input id="name" type="text" /></td> <td id="address">Address</td> <td><input id="address" type="text" /></td> <td id="phone">Phone number</td> <td><input id="address" type="text" /></td> <td id="country">Country</td> <td><input id="country" type="text" /></td> </tr> </table> <input type="button" onclick="Update();" value="Update" />
In the button click method we are calling the Update() method.
function Update() { var empLst = new Array(); $('#tblEmployees tr').each(function () { var obj = new Object(); var name = $(this).find("input").each(function () { if (this.id == 'name' && this.value!='') { obj.Name = this.value; } else if (this.id == 'address' && this.value != '') { obj.Address = this.value; } else if (this.id == 'phone' && this.value != '') { obj.PhoneNo = this.value; } else if (this.id == 'country' && this.value != '') { obj.Country = this.value; } }) empLst.push(obj); }); emps = JSON.stringify({ empLst: empLst }); $.ajax({ contentType: 'application/json; charset=utf-8', dataType: 'json', type: 'POST', url: '/Employees/UpdateEmployees', data: emps }); }
In this method we are looping through the rows of the table tblEmployees using the jQuery each() function.In the function passed to the each method we are creating a new javascript object using the following
var obj = new Object();
The objects we are creating in each method are added to a javascript array using
empLst.push(obj);
Finally we are passing this array using the jQuery ajax method to the action method.We are converting the javascript object to json object using the JSON.stringify() method as:
emps = JSON.stringify({ empLst: empLst });
We have defined the controller as:
public class EmployeesController : Controller { public ActionResult Home() { return View(); } public ActionResult UpdateEmployees(List<Employee> empLst) { //Perform operations on empLst return View(); } }
We are passing the following parameter to the UpdateEmployees action method which we are calling using the ajax() method
List<Employee> empLst
After the action method is called the empLst parameter will contain the list of employee objects which we had passed using json.
So while posting json data using jquery ajax in MVC we can get the objects in the action method without the need to manually map the posted values.

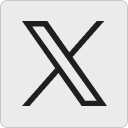
Very well put up. I am new to this field and was having hard time understanding AJAX call and all. Your post really made it easy.
Thank you.
Khyati , glad you found the article helpful.Thanks for the support and feedback.
This code is very very helpful and resolved my problem.
Thank you soooooooooo much….!
after the button click event i need to print the list of datas using jquery ajax method