jQuery includes several methods for implementing the ajax functionality in our applications.In the last post we looked at the jQuery get() and post() methods.These methods ,as per their names, makes GET and POST requests to the server.Behind the scenes these methods use the jQuery ajax() method.So it helps to understand the ajax() method which provides lot of different options for making asynchronous calls to the server.In this article we will also look into a jquery ajax method example in mvc.
The basic syntax of the ajax() method is
$.ajax({name : value ,name : value .....})
We can pass different key value pairs to the method in a javascript object.These name value pairs configures the asynchronous requests to the server and each key value represents some aspect of the asynchronous request.Some of the important values we can pass into the method are
url This key specifies the url to which we are making the request.So this is one of the most important key we need to pass to the method.Its important to realize that if we don’t specify this value then the request is made to the current page.In case of MVC the request is made to the action method for the view if we don’t specify this value.
success This key specifies the callback function which will be called if the request completes successfully.This method gets as parameters the data returned from the request,status of the request and also the XMLHttpRequest object .
error This key specifies the callback function which will be called if the request fails .This method gets the data returned from the error,status and the jqXHR object as parameters.
Below we are making a simple request to an action method using just the above key values.
$("#btnSubmit").click(function () { $.ajax({ url:"/Employees/EmployeeDetails", error: function (xhr, status, error) { alert(error); }, success: function (data,status) { alert(data); }, });
type Specifies the type of request to use for the ajax call.We can use HTTP methods POST, GET, PUT.Default value is GET which is used if we don’t specify the type value.
It’s important to understand that by default the ajax() method makes the GET request to the specified url. So in this case the request will succeed if we have a GET action method. If we need to make the POST request to the server we need to set the type value .
So if our action method is defined as below and we don’t specify the type value in the ajax method we will get a “Not Found” error response from the server.
[HttpPost] public ActionResult EmployeeDetails() { return View(); }
To call the above action method we need to add the key for type and set its value to “POST”.
type :"POST"
data If we have to pass the data to the action method using the ajax() method then we need to add the value for data key.So to pass the employee object as an action method parameter we can create a json object and set it as the value of the data key.
var Employee = { "name": "Ashish", "address": "AddrXYZ", "salary": "SalXYZ", "id": "idXYZ" } $.ajax({ data: Employee, contentType: "application/json; charset=utf-8", url:"/Home/EmployeeDetails", error: function (xhr, status, error) { alert(error); }, success: function (data,status) { }, success: function (data) { }, });
We need to have a model object with the same properties as the json object defined above.
public class Employee { public string name { get; set; } public int id { get; set; } public string salary { get; set; } public string address { get; set; } }
Now we can define our action method as
[HttpGet] public ActionResult EmployeeDetails(Employee emp) { return View(emp); }
When the request is sent to the action method the values that we have set for the javascript object in the ajax() method data key are are used to populate the Employee object of the action method parameter .
dataType This specifies the type of the data that our $.ajax() method is expecting back from the server.We are not required to specify this value most of the time as MIME type of the response is used by jquery to identify this value.
These were some of the commonly used values that we can pass to the $.ajax() method.
$.ajaxSetup()
There is a $.ajaxSetup() method in jquery which can be used to provide default values for the $.ajax() keys.
If we set the values for the $.ajax() keys in the $.ajaxSetup() ,then the ajax calls we make using jquery will use the $.ajaxSetup() settings so we don’t need specify them when making the call.So if we set the url using the below then we don’t need to specify the url in $.ajax()
$.ajaxSetup({ url: "HomeIndex" });
So if we now call the the $.ajax() method without any parameters it will send the ajax request to the url we have set above.

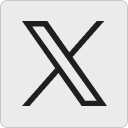
Leave a Reply