Numpy is a library in Python which is used for scientific computing.The main object provided by numpy is array.Array in numpy is know as ndarray or simply array.It is important to note that numpy array is different from Python array.
To create a numpy array use the following syntax:
Import the nump library:
import numpy as np
Create a numpy array
arrObj = np.array([1,2])
Now if you print the array you will get the following:
print(arrObj )
Output:
[1,2]
Few important things to remember about numpy arrays:
- Shape is the dimension of the array.In the above example it is 2.
- Size is the total elements in the array.So if the dimension of the array is x,y then its size will be x*y
Basic operations
If you define two numpy arrays as
a = np.array( [1,2,3,4] ) b = np.arange([2,2,2,2] )
the doing a+b will output the following array:
array([3, 4, 5, 6])
as you can see numpy array automatically adds elements of the two arrays.
Creating from a List
You can create a numpy array from list by passing list as the argument:
lst= [1,2,6,7] arrObj= np.array(lst)

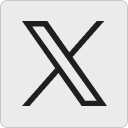
Leave a Reply