Python is an object oriented language.You can create class and their objects in Python.You can write OOP using Python but it is not necessary.In fact for for simple programs you will just write functions.
You define a class in Python using the class keyword followed by the name of the class.Once you have created a class you can create new objects of that class.The objects of the class consists of
attributes and fucntions defined by that class.
To define a new class implement the following steps:
- declare a class header by specifying class name after the class keyword followed by colon(:) as:
class Employee: define function within class as: class Employee: def fetchEmpDetails(self): print("employee name")
In the above function called fetchEmpDetails we are just printing the value employee name of the class instance variable
2.We can modify the above class to print empNo attribute.We will access the empNo instance attribute using getter and setter as:
class Employee: def __init__(self, empNo = 0): self.set_empNo(empNo) def get_empNo(self): return self._empNo def set_empNo(self, value): if value < 0: raise ValueError("empNo below 0 is invalid") self._empNo = value
Now if you call the get_empNo() getter then value “15” will be printed.
emp = Employee(15) print(emp.get_empNo())

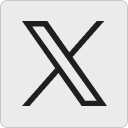
Leave a Reply