Dictionary in Python like list is a collection of objects.Unlike lists ,items in dictionary are accessed by key.Similar to lists ,items can be added or removed from dictionary.While the items in a list are sorted in a specific order ,the items in a dictionary are not sorted.The size of dictionary is not fixed ,items can be added or removed from the dictionary.
Creating a dictionary
To create a dictionary you specify key,value pairs.The keys and values are separated by colon.
Dictionary is declared using the parenthesis as:
dict = {'item1': 1, 'item2': 2}
In the above dictionary there are two elements.The key of first element is ‘item1’ and key of the second element is ‘item2’ .
once dictionary is declared you can modify the dictionary by using the key as:
dict['item2']="two"
You can get the length of the dictionary by using the len() function as:
dict.len(dict)
The items in the dictionary can be retreived by using the items method as:
dict.items()
Removing item from a dictionary
You can delete item from a dictionary using the del keyword as:
del sampleDict['2']
looping through the items in the dictionary
for x in sampleDict: print('x=%s'%(sampleDict[x]))

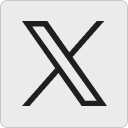
Leave a Reply