TypeScript adds new useful features to JavaScript. One such feature is type system in TypeScript. In JavaScript types are dynamic,this means that variable are not of
any specific type.So a variable can be assigned values of different types during its lifetime.
So the variable a below can be assigned a numeric value:
var a=1;
and later on it can be assigned a string value
var a="1";
So JavaScript is not strongly typed language.This means that types in JavaScript are not known at compile time and there are no strict type rules.
Static typing
TypeScript is a statically typed language.This means that the types are known at compile time.So for example if we declare a variable as
var a : number = 1;
then the type of ‘a’ is known at compile time and will remain same throughout its lifecycle.
Another way we can declare variable the variable in TypeScript is:
var a= 1;
This declaration is similar to the above one ,but the type of ‘a’ which is number is determined automatically by typescript determines that ‘a’ is of number type based on the value which it is assigned.
Also TypeScript is a strongly typed language which means that TypeScript has strict type rules.
There are two main types in TypeScript
- Primitive type The main Primitive types in TypeScript are:
- Number Represents number as well as decimal values.
- String Represents string values enclosed in single or double quotes.
- Boolean Represents true-false values.
- Enum Numeric constant values
- undefined Represent a value which is assigned to a variable which is not assigned a value.
- null Object which is currently not having any value.
- Any Represent an untyped variable which can be assigned any type of value.
- Object types
An important type of variable in typescript is declared using the keyword ‘any’.This type of variable behaves just like a variable in JavaScript.
So if we declare a variable as:
any a=1;
then the variable ‘a’ is not of any specific type.So we can also assign a string value to ‘a’:
any a='temp value';
Object types are types such as classes and interface.
Declaring custom types
Class
We declare a class by using the keyword class followed by the interface name:
class Employee{ public name: string; public id: number; }
Interface
We declare an interface by using the keyword interface followed by the interface name:
interface IAdd{ x: number; y: number; }
Enum
Enum is a group of named numeric values.Enum in TypeScript is declared by using the keyword enum followed by the enum name:
enum Departments{ Administration, Finance, HR, Sales }

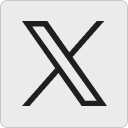
Leave a Reply