TypeScript has the concept of modules.Here we will see how to declare modules in TypeScript.
When working on any application it helps to keep the code organized.Keeping the code organized provides the following advantages:
- Code is easier to locate since similar functionality is part of a single module
- Code is not duplicated since required code can be exported from an existing module
- It is easier to update and maintain an application since the application is composed on independent modules
Declaring Modules in TypeScript
Modules in TypeScript are similar to modules in other languages such as c#.We put the required types such as classes and interfaces in a module.
In typescript there are two types of modules:
Internal modules Used for organizing our application.We segregate the types in our application into different modules.This helps with managing the application.This is similar to namespaces in c#.
External modules An external module is defined in a single JavaScript file and loaded when required using a module loader.
External modules are frequently used when we are working in Angular 2 applications.
External modules
External modules are different from internal modules.While internal modules can be directly consumed external modules are loaded using a module loader such as RequireJS. External module is defined in a separate TypeScript file.
If we want to expose a type defined in a Module then we use the export keyword when declaring a type:
export Type TypeName {
}
For example we can declare a type called Employee as:
export class Employee { name: string; rollNo: string; standard: number; }
The file itself represents an external module.
So if we want to define a module called rootmodule then we need to create a file called rootmodule.
Then if we want to import the members defined in the rootmodule we can use the import statement.Assuming we have declared the above employee class in a file called employeemodule.ts we can consume the external module by using the import statement as:
import { employee} from ‘./employeemodule’;
Once we declare an instance of employee class we can use it like any other typescript variable:
public emp:employee;
Internal Modules
We declare an internal module by declaring an identifier following the Module keyword and enclosing the types in curly braces.When declaring the types in the module we
place the export keyword to make the types accessible outside the module.
module ModuleName{
Type declarations…
}
Module Shipping {
In the following example we are declaring a module called Organization
module Organization { export class Employee { constructor(public name: string, public Id: string, public department: Department) { } } export class Department { constructor(public DepartmentName: string) { } }
We can create objects of the above classes in our code as:
var dept = new Organization.Department("AD"); var emp = new Organization.Employee("ashish", "001", dept);
The classes Organization and Department are accessible in our code because we have exported these classes.
It is important to declare the members of the module using the export keyword otherwise the members will not be visible outside the module.
For a brief overview of TypeScript please refer Getting started with TypeScript

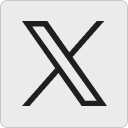
Leave a Reply