TypeScript is a strongly type language.This means that variables and expressions are type checked at compile time.But if required we can turn off this behavior.
Variable declaration in JavaScript
In JavaScript variables are not strongly typed.This means that following statements are valid:
var var1 = 'string value'; var1 = 64;
We can assign different types of values to the same variable in JavaScript.
Variable declaration in TypeScript
Declaring variables in TypeScript is similar to variable declaration in JavaScript.But there is one important difference.Unlike JavaScript ,TypeScript is a strongly typed language.
In TypeScript when we declare a variable we assign a type to it.For example we declare a string variable as:
stringValue: string = "some value";
The variable stringValue above is declared as string using type annotation.
Similarly we can declare number and boolean values as:
boolValue: boolean = false; numericValue: number = 6;
If we required we can opt out of the strict type checking by using the “any” keyword.If we declare a variable as any type then it is not type checked at compile time.
This behavior is similar to the behavior of variables in JavaScript.
let tempVariable: any = 53; tempVariable = "string value";

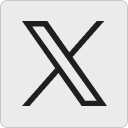
Leave a Reply