Interface means how one entity interacts with other.For example a class may have some internal implementation.But its only the interface which is visible to the consumers of the class.
Unlike other types such as classes which provide the actual implementation in application ,interfaces just describes other types.We can define interface in TypeScript which can be useful in several scenarios:
As method parameters
We can declare function parameters by using interfaces.Object of any type can be passed as an argument to such a function.A distinguishing feature of interfaces in TypeScript is ducktyping. Ducktyping means that the type checking is based on the shape of a variable.For example if a variable has the same structure as Interface then that variable can be used as interface variable.
For accessing complex objects
A common scenario is calling a method that defines an object argument have multiple properties.To call such a method in normal JavaScript we need to create an object and set all the properties. Since object is expected by the method and object should define all the properties ,so even if we don’t require all the arguments we still need to pass these .Using optional properties in Interfaces we can assign only the required parameters and ignore the rest.
A common example is jQuery $.ajax method.The signature of this method is:
$.ajax(url[, options])
We pass only the required arguments to the options object.TypeScript optional properties are useful for defining such functionality.
For accessing external APIs
While using external code we may find it difficult to understand the different methods.In such a scenario we can just pass the interface variables.
Its important to remember that Interfaces in TypeScript is just a compile time construct.Once TypeScript code is compiled ,interfaces doesn’t exists.
Defining Interface in TypeScript
We can declare an interface as:
interface IMessenger{ message: string; }
This will define an interface called IMessenger which defines a single property named message.Now IMessenger interface can be used anywhere to declare a variable.
We can use it to define function argument:
function Hello(msg: IMessenger) { console.log(msg.message); }
Also we can define a variable of IMessenger type:
var coolMessenger : IMessenger;
In the following example we are declaring a variable called genericMessenger. We are then passing this variable to Hello method.Since the strucuture of the variable genericMessenger is same as IMessenger interface it can be used as IMessenger type.
let genericMessenger = {Format: "HTML", message: "Hello world"}; Hello(genericMessenger);
Declaring optional property in Interface
We define optional properties in interface using the ? after the property name.In the following example we declared interface called Person which will represent a person.Since every person has a name and age these are declared as normal properties.But driving license and passports are not held by every person.So these can be declared as optional properties.
interface Person { name: string; age: number; drivingLicenseNo?:number; passportNo?:number; }

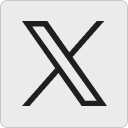
Leave a Reply