Looping is an important construct of any language.Looping means to repeatedly display a group of elements.Loops in TypeScript in TypeScript are useful when working with a collection.
For Each
For displaying elements in an array a useful method is forEach ().It executes the code for every element in array.
So if we pass a method to this method then it is called for every element in the array.
It is like foreach statement in other languages.So if we have an array containing names then we can display alert for
every element in the array using forEach() method.
class Utility { Print(element) { alert(element) } } let utility = new Utility(); var employees:string[]=[]; employees = ["Marc","John","Ram"]; employees.forEach(emp=>utility.Print("Employee Name:"+emp));
the above code will display alert message for every name in the collection.
We can also use TypeScript forEach() method with a TypeScript array containing objects. forEach loop for
array of objects can be used for evaluating each object.In the following example we are just displaying each object’s properties
class Utility { Print(element) { alert(element) } } class Employee{ constructor(public Name: string, public Salary: number, public EmpId: number) { } } let utility = new Utility(); var employees:Employee[]=[]; employees[0] = new Employee("Marc", 5000, 1); employees[1] = new Employee("John", 5000, 2); employees[2] = new Employee("Ram", 5000, 3); employees.forEach(emp => utility.Print("Employee Name:" + emp.Name + "Employee Salaray:"+emp.Salary+ "Employee EmpId:"+emp.EmpId));
For in loop
for in loop is used to iterate over a collection of values.If there are 5 elements in a collection then the loop will be executed 5 times.
Its syntax is as:
for (var element in collection) {
//statements
}
In the following example an array is declared having 5 elements.The elements in the array are iterated using the for in loop as:
var coll: string[]; coll = ["a","b","c","d","e"]; for (var alphabet in coll) { alert(alphabet); }

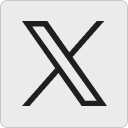
Leave a Reply