Named and Default valued parameters in TypeScript are useful features which can help us remove code redundancy when we use method overloads.
Since TypeScript is a strongly typed language so it is type checked at compile time.If a method has four parameters then we need to provide 4 arguments ,otherwise TypeScript compiler will throw error.Optional and default valued parameters helps to resolve this issue.
Optional Parameters in TypeScript
When we define a function in TypeScript we provide list of parameters along with the type of each parameter.When we call the function the number and type of argument should match the number and type of arguments.If we don’t follow this then we will get compilation error.
For example if we declare the GeFullName function as:
class Calculate{ Add(a: number, b: number): number { return a + b; }
then we need to provide two arguments of string types otherwise we will get an error.If we call the above function as:
var obj = new Calculate(); obj.Add("1", 2);
the compiler will complain about the mismatch between the argument and the parameter.
Also if we call a method by omitting a parameter we will get a compilation error
In the above example compiler is displaying error message because by default all the method parameters are required.We can fix the above error by making one of the parameters as optional.To make a parameter optional we append the question mark ? after the parameter name
Add(a: number, b?: number): number { return a + b; }
Now we can call the above method by providing only a single argument.
obj.Add("1", 2);
Default valued parameters in TypeScript
Suppose we want to method parameter to use a default value when a value is not supplied for an argument.In such a scenario we can define a default value for the method parameter.
In the following example we have defined parameter with default value
let Add =function Add(a: number, b: number=2): number { return a + b; }
Now if we call the above method without providing any value for the parameter b then alert will display the value 3.This is because b has a default value of 2.
alert(Add(1));

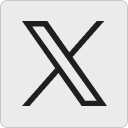
Leave a Reply