Binding combo-box to some data means just setting the data context of the combo box to a list of values.In the case ItemsControl such as combo-box the ItemsSource property needs to set to a list or collection of values.
Binding ComboBox using MVVM in WPF
The source for displaying list of values in the combo-box needs to be populated with values in the ViewModel.Below EmployeeViewModel is declared which defines the Employees property which will be used to populate the combobox.
namespace SimpleMVVM { class EmployeeViewModel { public IList<Employee> employees = new List<Employee> { new Employee{Name="Marc",Id=2}, new Employee{Name="John",Id=2}, new Employee{Name="Anthony",Id=2}, new Employee{Name="Amit",Id=1}, new Employee{Name="Rakesh",Id=2} }; public IList<Employee> Employees { get { return employees; } set { employees = value; } } public ObservableCollection<Employee> LstEmployees= new ObservableCollection<Employee> { new Employee{Name="Marc",Id=2}, new Employee{Name="John",Id=2}, new Employee{Name="Anthony",Id=2}, new Employee{Name="Amit",Id=1}, new Employee{Name="Rakesh",Id=2} }; } }
XAML
Combox box to display list of employees can be declared in XAML as:
<ComboBox cmbEmployees Height="20px" Width="100px" ItemsSource="{Binding Employees}"></ComboBox>
Here the ItemsSource property is bound to the Employees property in the source object.
The datacontext will be set the codebehind as:
public MainWindow() { InitializeComponent(); Employees emp = new Employees(); this.DataContext = emp; }
If you now run the application you can see the combo-box displaying some weird values.It is not what you expected to display in the combobox. To display the properties defined in the Employees property you need to set the following properties of the combo-box:
SelectedItem This represents the currently selected item in the combobox.If this value is set then the combox will display this as the selected value.This represents
the current entity in the combobox.Once the user selected item in the combobox ,the selected item could be retreived using this property
DisplayMemberPath This is the property which needs to be displayed in the combobox.As the object which is bound to the combobox could contains many proprties ,the
property which needs to be displayed in the combobox needs to be specified.DisplayMemberPath is used to specify this property.
Currently the combobox will display the list of employee names.When selected employee name is changed in combobox nothing happens.To make the currently selected value in combobox reflect in ViewModel make the following two changes.
1.Add a property in the ViewModel representing the currently selected value in the combobox:
private Employee _employee; public Employee SelectedEmployee { get { return _employee; } set { _employee = value; } }
2. Set the UpdateSourceTrigger property of the combobox as PropertyChanged event.
<ComboBox x:Name="cmbEmployees" DisplayMemberPath="Name" Height="20px" Width="100px" SelectedItem="{Binding SelectedEmployee}" ItemsSource="{Binding Employees,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}"></ComboBox>
Now the SelectedEmployee property will be set as the current item selected in the combobox

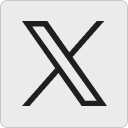
Leave a Reply