Commands in WPF are used for executing logic in response to different actions.Here we will be Implementing ICommand in WPF using MVVM.
Commands are invoked as a result of different actions.There could be different types of actions such as delete,copy and save.In a normal application that does not use commands the logic which gets executed as a result of an action is tightly coupled to the action.So if the user performs an action such as copy then there is specific logic for copy action which is specific to the copy action.If any other action such as cut wants to use the same logic then the logic needs to be duplicated in the event handler for cut action.
Command is used in WPF to decouple the action invoker such as button from the object which would handle the logic executed as a result of invoking the action.There are several advantages of using this approach:
- As the action invoker is loosely coupled with the action handler ,the same logic could be used by different actions.This prevents the duplicacy of code.
- Command indicates whether it can be invoked through the CanExecute method.So if this method returns false then command could not invoked.Developer can disable the control such as button if CanExecute return false.
Implementing ICommand interface
Comamnd in WPF is a class which implement the ICommand interface.ICommand has the following memebers which needs to be implemented in the implementing class:
- Execute is a method which contains the actual logic for command handling
- CanExecute is a method which returns boolean value indicating whether the command can be executed or not.
- CanExecuteChanged is an event.
When implementing command normally Execute and CanExecute methods are implemented.
In the following example we will implement a simple command using MVVM pattern.In the previous MVVM example we have implemented a basic ViewModel.
We will add new command called UpdateCounterCommand.This command will implement the CanExecute and Execute methods.In the CanExecute method we are just returning true.
In the Execute method we are just updating the counter variable
public class UpdateCounterCommand : ICommand { static int counter = 0; public event EventHandler CanExecuteChanged; public bool CanExecute(object parameter) { return true; } public void Execute(object parameter) { counter++; } }
In the EmployeeViewModel property of type UpdateCounterCommand is defined.
UpdateCounterCommand _updateCounterCommand = new UpdateCounterCommand(); public UpdateCounterCommand UpdateCounterCommand { get { return _updateCounterCommand ; } }
In the XAML Command property of button is set to the UpdateCounterCommand command.
<Button Content="Add" Command="{Binding Path=DeleteEmployeeCommand}"></Button>

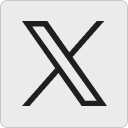
Leave a Reply