In every application there are a sequence of method calls.A method may call second method which may call the third method.
This sequence of method calls is known as the call stack.In .NET this call stack is represented by the StackTrace class.
We can access the StackTrace information for understanding the execution of the program and for other debugging purposes.
In the following program method Method1 calls Method2 which calls Method3.
class StackTraceDemo { public void Method1() { Console.WriteLine("Method1"); Method2(); } public void Method2() { Console.WriteLine("Method2"); Method3(); } public void Method3() { Console.WriteLine("Method3"); } }
We are calling the method Method1 from the Main() method as
static void Main(string[] args) { StackTraceDemo obj = new StackTraceDemo(); obj.Method1(); Console.ReadLine(); }
On executing the above program we get the following output
We were able to identify the sequnce of method calls from the Console.WriteLine() methods.But using the Console.WriteLine() statements might not be always possible.So we use the StackTrace class for debuging the application.StackTrace class in C# represents a stack of method calls.To access a specific method call we can use the StackFrame class.
So if we comment the Console.WriteLine() methods in the above example and use the stack trace class we will be able to get detailed information about the method calls
class StackTraceDemo { public void Method1() { //Console.WriteLine("Method1"); Method2(); } public void Method2() { // Console.WriteLine("Method2"); Method3(); } public void Method3() { //Console.WriteLine("Method3"); StackTrace stackTrace = new StackTrace(); // get call stack StackFrame[] stackFrames = stackTrace.GetFrames(); // get method calls (frames) // write call stack method names foreach (StackFrame stackFrame in stackFrames) { Console.WriteLine(stackFrame.GetMethod().Name); // write method name } } } class Program { static void Main(string[] args) { StackTraceDemo obj = new StackTraceDemo(); obj.Method1(); Console.ReadLine(); } }
We get a similar output when we use the StackTrace to print the sequence of method calls.

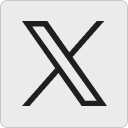