Aggregate functions are applied to each element in a sequence and finally returns a single aggregated value.For example sum() function is applied to the first,second element to calculate their sum.This sum is then added to the third element to calculate the sum of first three elements.Finally the total sum of all the elements is calculated and returned.
Some of the aggregate operators are:
- Sum
- Count
- Average
- Min
- Max
Sum Sum operator is applied to the values in a sequence and calculates a single value which is the sum of all the values in a collection.
In the following example we have a collection of students.Each element in the collection is represented by an object of Student class.
class Student { public String Name { get; set; } public int TotalMarks { get; set; } //other properties }
The students are added in the collection:
List<Student> lstStudents = new List<Student> { new Student{Name="Marc",TotalMarks=774}, new Student{Name="John",TotalMarks=789}, new Student{Name="Amit",TotalMarks=888}, };
The sum of the scores of all the students can be calculated by using the sum function as:
lstStudents.Sum(x => x.TotalMarks);
We can write the above Sum() operator as:
int sum = lstStudents.Select(x => x.TotalMarks).Sum();
Note that we have passed the lambda expression to the Select() operator instead of Max().We can use the similar method overloads for each of the aggregate operators.
Max Many times when working with numeric values we need to find the maximum value from a collection of values.Max operator can be used in such a scenario to find the maximum value from a collection of values.Continuing with the example of collection of students ,the student having maximum score can be found as:
int max = lstStudents.Max(x => x.TotalMarks);
Above Max() function can also be written as:
int max= lstStudents.Select(x=>x.TotalMarks).Max();
Min Sometimes it is required to identify an element having a minimum value in a collection of elements.Min operator is used to find a minimum value from a collection of values.
In the following example we have a collection of days and temperatures.
Dictionary<int, int> tempratures = new Dictionary<int, int>(); tempratures.Add(1, 15); tempratures.Add(2, 18); tempratures.Add(3, 20); tempratures.Add(4, 16); tempratures.Add(5, 12); tempratures.Add(6, 19); tempratures.Add(7, 17); tempratures.Add(8, 26); tempratures.Add(9, 16); tempratures.Add(10, 19);
The minimum temperature can be identified as:
int min=tempratures.Min(x => x.Value);
Average To calculate the average of numeric values in a collection we can use the Avg operator.
Continuing with the previous example of days and tempratures ,average temprature can be calculated as:
double avg = tempratures.Average(x => x.Value);
Count This returns the total number of elements in a collection.
Some of the main points about aggregate functions in LINQ are:
- Aggregate queries are executed immediately unlike other operators which are executed when the collection is iterated.
- Aggregate queries typically return a number instead of a collection.

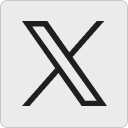