Class is a reference type.We can define custom classes ,apart from the classes provided by the framework.Though class is one of the most commonly used data type ,sometimes we need to define a custom value type.The main reasons for defining a custom values type rather than a reference type are:
- Value types are efficient in terms of memory as they are stored on the stack.Also extra memory is required for storing the object reference.This can be a big overhead in some cases.
- They are more efficient when the memory is garbage collected.
- If we need to define a data type to store some numeric values struct is more efficient.
This is where structs are useful.We define structs similarly to how we define classes.
struct Employee { public string Name{get;set;} public int Id{get;set;} public string Department{get;set;} public string Address{get;set;} }
Like classes struct can define function and data members such as:
Methods
Fields and Properties
Constructors
Following are some of the characteristics of structures
- We can not declare a parameterless constructor if we are using the version of C# prior to C# 6.0.
- Structure can not inherit from other structure or class.Though a structure can implement interfaces.
- We can create an instance of a structure without using a constructor.If we don’t use the constructor then the fields are not initialized.
- When we create an instance of a Structure it is allocated on the Stack.Class objects are allocated on the Heap.
- Numeric Value types such as int are implemented as structures.
- Structures in C# are used to group related values and have less memory overhead in many scenarios.They provide a good to alternative to class if we want to group related numeric variables.

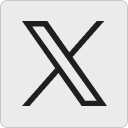