Angular 2 application consists of a collection of components.Components in Angular 2 application need to interact with other components in the application.
Angular 2 provides two useful property decorators for interaction between components.
Input and Output decorators are used to pass data into and out of the component.We attach the decorators to the component properties.
- @Input is attached to a property that can be used for property binding
- @Output is attached to a property that can be used for event binding
To identify a property as input we annotate the property with @Input() decorator.
@Input() name : string;
We can alias the property name.Aliasing property means that property will be accessed using the alias instead of actual name.
For example in the following example name property will be accessed using the identifier employeeName instead of name.
@Input('employeeName') name : string;
Similarly to identify a property as output we annotate the property with @Output() decorator.
In the below example we are declaring a property as output.@Output decorator is used with EventEmitter datatype.
@Output() sendInfo = new EventEmitter<string>();
We can alias @Output() property which will allow us to use different name when binding the property
import { Component, Input } from '@angular/core'; @Component({ selector: 'sample-component', template: '<div>{{name}}</div>' }) export class SampleComponent { @Input() name : string; constructor() {} } <sample-component [name]="employeeName"></sample-component>

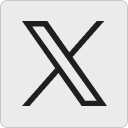