Following are some of the important Angular 2 Interview Questions and Answers
Describe important features of Angular 2?
Angular 2 is a JavaScript framework for developing web applications.Since it is a complete rewrite of Angular 1.0 ,so learning AngularJS 1.0 is not required for creating Angular 2 applications.
It is well suited for developing mobile applications unlike AngularJS 1.0 which was suitable for building desktop applications.It is also much faster than Angular 1.0.
It supports the modern browsers as well as the older browsers.Applications are better structured than previous versions of Angular.It supports server side rendering for faster rendering of views even on slow devices such as mobile.The size of Angular 2 library is smaller compared to previous versions.Also Angular 2 applications use ahead of time compilation which makes them faster.
What are the advantages of Angular 2 ?
Angular 2 being a complete rewrite of AngularJS provides several benefits over AngularJS.Some of the advanatages of using Angular 2 are:
- Cross platform Angular 2 applications can run on different platforms such as Mobile,Desktop.
- Server Side Rendering improves the page load time.This is specially useful for devices such as Mobiles.This is also better for SEO.
- Modular Applications developed using Angular 2 are modular as they are developed using TypeScript.TypeScript applications are organised using components.This results in better organisation of application.
- Lightweight Angular 2 applications are lightweight and faster compared to AngularJS.
Better performance because of these reasons:
- Better change detection.
- Ahead of Time compilation (AOT) improves rendering speed.
- Lazy Loading.
- TypeScript can be used for developing Angular 2 applications.
- Better syntax and application structure.
Which languages are used for developing Angular 2 applications?
Angular 2 applications can be developed in any of the following languages:
- Typescript Prefered Language for developing Angular 2 applications.
- JavaScript
- Dart
Though any of the above languages could be used for developing Angular applications but Typescript is the preferred language for developing Angular 2 applications.. As Angular 2 is written in TypeScript so it is preferable to develop Angular 2 applications in TypeScript.
We don’t have to worry about the JavaScript or ECMAScript version as its the compiler’s responsibility to manage the version issues.
What are Components?
A component is a building block of Angular 2 application.Angular 2 application is created as a tree of components.A component is declared by using @Component() decorator function.To develop Component you first create a class and then decorate it with the @Component() decorator.
@Component({ selector: 'first-component', template: `<p>Hello from first component</p>` }) export class FirstComponent { }
When we declare Component we define metadata for component.In this example we have defined selector and template metadata for the FirstComponent component.
Though we have defined inline html above ,we can declare html in a separate html file and use the the templateUrl property in the Component decorator:
templateUrl: './app.component.html'
Similarly we can define css in a separate css file and link to it from component as:
styleUrls: ['./app.component.css']
Also we usually use repository in a component class which is injected in the constructor of the component as:
constructor(private employeeRepository: Repository) { }
What is a Module?
Any application consists of various functionalities for different uses.For example there could be a functionality related to fetching employees from a database or a functionality for displaying those employee
details.If you have several features in your application then you can break those into different Angular modules to create an application which is easier to maintain.Modules are common in most of the languages.
Angular modules serves a similar purpose for creating a loosely coupled application.Angular modules are defined using the @NgModule() decorator.You use @NgModule as:
@NgModule({ providers: [EmployeeRepository], }) export class FetchEmployee{ }
So Modules segregates application functionality.Module can consist of collection of components,directives and services.Every application has one root module which is bootstrapped to launch the application.Angular app usually consists of various modules for different functionalities.These are feature modules.
Angular modules are created using the NgModules() decorator function.
Angular application consists of a root module defined in app.module.ts.This defines the all the modules used in the application.
What is a JavaScript Module?
Often we need to define reusable logic in our application.
JavaScript ES2015 provides a way to define reusable logic using modules.Functionality which you want to reuse across your application you define in a module.So JavaScript file is a module.For example you can define a JavaScript file called Utility.js as:
export function displayTime() { d=new Date(); alert(d.getTime()); }
then you can reuse the above function in a separate JavaScript file as:
import {displayTime} from './Utility.js'; displayTime();
What is app.module.ts file?
Application consists of different functionalities for different things.Any specific functionality in contained in an Angular module.
Angular module is defined using the NgModules decorator.
An angular application consists of a root module called AppModule which is defined in a file named app.module.ts.This module is
responsible for launching or bootstrapping Angular application.
What are Angular directives?
Directives are instructions which are specified in DOM. They add behaviour to existing HTML markup.
The different types of directives in Angular are :
- attribute directives Attached to an element and modifies its properties and appearance.An Attribute directive changes the appearance or behaviour of a DOM element.
- structural directives Modifies the HTML code.
Component is also a directive that has both view and behaviour
What is a Service?
Service defines functionality which could be shared by different components.
You define a service by annotating a class with the @Injectable() decorator as:
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class TestService { constructor() { } }
Explain dependency injection in angular?
DI framework takes care of dependencies in Angular application.Angular DI framework is based upon providers, injectors, and tokens.
Injector registers all the dependencies using the providers.Suppose we have a class called class1.Now instead of creating he instance of Service1 we can pass it using the Angulars dependency injection as:
class class1 { constructor() { let svc1=new Service1(); } }
instead of writing the above logic we inject the service through the constructor:
class class1 { constructor(svc:Service1) { let svc=new Service1(); } }
Now for the service to be passed or injected using the constructor it has to be injectable or decorated using the @injectable() decorator.
we can declare a class using the Injectable decorator as:
@Injectable() class Service1{ }
What is a Template?
Component represents both the logic as well as the view for that logic.View of a component is declared by using the template.It is the template which is rendered.
We can define template in line in the component template metadata property:
@Component({ selector: 'hello-component', template: '{{Hello}}' })
We can also define templates in separate html file and use the templateUrl property in component:
@Component({ selector: 'hello-component', templateUrl: './hello.html' })
What is the use of constructor in component?
constructor is a function which is called before any life cycle hook is called.It is called for injecting application dependencies.In the following example a service called ProductsService is being injected into the constructor.
class SimpleComponent { products:Array<any>; constructor(private productsService: ProductsService) { this.products = productsService.getProducts(); } }
Which Data bindings which are supported in Angular 2?
Interpolation In interpolation binding we specify the binding using expressions
<h2>{{employee.name}}</h2>
Property Binding In property binding we bind the custom property using square brackets
<h2 [innerText]="employee.name"></h2>
Event Binding In event binding we enclose the event name in parenthesis and assign the event handler method to the event:
<button (click)="gotoDetail()">View Details</button>
Two-way Binding In Angular 2 we define two way binding as:
<input [(ngModel)]="employee.name"/>
Input and output decorators in angular?
These are useful when we have child components.
Input() decorator
When we want to pass value from parent to child then we declare input property in child:-
@Input() inputProp: string;
Output() decorator
Now if the child wants to pass some value to the parent component then it declares output property.This property is of type EventEmitter:-
@Output() outputProp = new EventEmitter<string>();
now in the parent component’s template we can declare the child component as:
<child-com (inputProp)="processMessage($event)"></child-com>
How Angular 2 application is launched?
There is a single root module in every Angular application.Angular application is launched by bootstrapping this root module.
What is Angular cli?
The Angular CLI is a tool which helps in the development,testing,build and deployment of the application.You can install the CLI using npm:
npm install -g @angular/cli
- Generates new project structure with required files instead o manually creating those files
- It can generate specific components, services, routes, and pipes
- It serves a localhost development server which is used in development
- It sets up Karma for running unit tests
For generating a new app we use:
ng new myapp
to serve the application
ng serve
to see all the available commands
ng --help
What is router-outlet?
The route which is matched by the router is used display the component.The template defined by the component is displayed in an area defined by the router-outlet.You declare router-outlet in a view as:
<router-outlet></router-outlet>
What is Angular Module or NgModule?
Angular module is class decorated with the @NgModule decorator function.
Its a decorator function which has one argument ,a metadata object with properties describing the module.
Some of its important properties are:
- declarations views which belong to this module.
- exports declarations which are visible in the components of other modules.
- imports other modules whose classes are needed in this module.
- providers services which are provided by this module
- bootstrap This property is set by the root module
@NgModule({ providers: list of providers, exports:list of components, imports:list of components })
What is package.json?
The package.json file is core to the Node.js and is also part of the Angular project.It consists of metadata about the project such as the name and version of the project and any project dependencies.The dependencies are the modules on which the project depends.
You can create a package.json file by using the npm command as:
npm init
Following are the important contents of example package.json file:
"name": "CodeCompiled", "version": "1.0.0", "description": "SamplePacakge", "author": "User", "dependencies": { }, "devDependencies": {}, }
dependencies and devDependencies specifies the modules which are required by your application.devDependencies section specifies the dependencies that are required only in the development.
What is a tsconfig.json file?
TypeScript is a language in which Angular 2 applications are written.It is a superset of JavaScript.Since browsers understand only JavaScript so TypeScript code need to compiled to JavaScript.This is done by the TypeScript transpiler. Using tsconfig.json file we specify the settings for the TypeScript transpiler. Main property in tsconfig.json file is compilerOptions property which is used to specify options to the TypeScript compiler.
Difference between promise and observable?
Promise returns a single value while observable returns multiple values
The calling function calls the asynchronous function which returns a promise.The calling function specifies what should happen when the calling function gets the promise object:
objectPromise.then(value => console.log(value));
If we have single values then we use promise objects.
Observable is also used for calling a function asynchronously but the calling function can return multiple values:
observableObject.subscribe(value => console.log(value));
So if your asynchronous function returns multiple values then you use observable and if it returns single value then you use promise.
New features in Angular 8 ?
Differential loading This improves performance as the appropriate bundles are loaded for the browsers,for browsers that support ES2015+ and for older browsers that support the ES5.
Dynamic imports for lazy routes Improves TypeScript and linters
CLI workflow improvements ng build, ng test and ng run are extended to 3rd-party tools.
How can you do Angular 7 to Angular 8 upgrade?
ng update @angular/cli @angular/core
Check which version of Angular you are using?
ng --version
this command will list the current version of angular and the current version of cli
Generate component and service using cli?
component:
ng generate component <component_name>
service:
ng generate service <service_name>
directive:
ng generate directive <directive_name>
What is lazy loading in Angular2?
Angular 2 application is a collection of modules and components.There are two ways we can load Modules:
- Eager Module loading Loading module at application startup
- Lazy loading Loading Module only when required
Module which is required can be loaded instead of loading all the modules at application initialization.This has the obvious advantage of improving the application startup time.
We enable lazy loading in Angular 2 by using the loadChildren property in route
{ path: 'URL', loadChildren: 'modulePath#ClassName' }
What is LifeCycle hook?
Each Angular component has a life cycle which is composed of different phases.You can handle methods to implement functionality when certain lifecycle event happens
- ngOnInit when component’s properties have been initialized
- ngDoCheck for implementing functionality related to change detection
- ngOnDestroy when component is destroyed
What is @Injectable ?
In Angular a service is a class decorated with the @Injectable decorator.
What is AOT compilation?
AOT compilation stands for Ahead Of Time compilation, in it angular compiles components to native JavaScript and HTML during the build time instead of runtime.
This drastically improves the performance of the Angular 2 application.With Just in time compilation ,the compilation happens on the users browser at runtime.In the case of Ahead of time compilation ,the application is compiled and optimized at the build time instead of run time.So this improves the rendering of the application UI.This approach should be used in production builds.

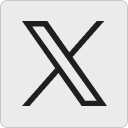