Different languages provides for to work with a collection of elements.Similarly when developing User interface sometimes we need to repeat a group of elements.For example there are can be a list of employees which we need to display.To implement this we need to loop over all the elements in a collection and add them to DOM one at a time.
If we have to implement this manually then it will be a tedious and error prone process.In Angular 1 we have ng-repeat directive to work with a collection of items.It creates a new template for every item in the collection.
Angular 2 doesn’t have ng-repeat but has a new directive ngFor for working with a collection of items .If we use this directive then the template which we provide will be instantiated for every item in the collection.
We use it as
<li *ngFor="let item of items; let i = index"> {{i}} {{item}}</li>
above items is the collection which contains the items that we want to display.item represents a single element in the items collection.
We are displaying item here.So if item contains integer values from 1 to 5 then it will display the numbers from 1 to 5.
In the following example we have implemented a component which consists of a collection of employees.We are setting the employees array in the constructor.
In the template of the component we are just looping over the array of array and displaying the elements.
import { Component } from '@angular/core'; @Component({ selector: 'example-component', template: `<ul><li *ng-for="#employee of employees">{{employee}}</li></ul>` }) export class ExampleComponent{ employees: string[]=[]; constructor() { this.employees = ['Marc', 'Amit', 'Tony', 'Greg']; } }
Above component will display the employees in a list.
ngFor provides several variables which we can use in the template.Following variables can be used in the tempalte created using ngFor
- Index represents current iteration.
- first true or false value which indicates whether this is first item in the collection.
- last true or false value which indicates whether this is last item in the collection.
- even true or false value which indicates whether the index is even.
- odd true or false value which indicates whether the index is even.

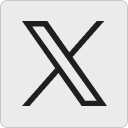